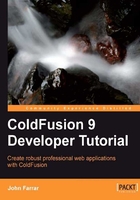
Our first object
Our first object is a generic model of a product. There are things that different products have in common. This object will be a combination of the common attributes and methods. The difference is that this is a computer object. Yet, we will do things in ways that reflect the real world. Our product object has attributes and methods. We will map them out here below. Remember, this will all make more sense as we work through this chapter. Along the way, you will get an understanding of both how CFCs work and how to interact with a database.
Product (object)
The attributes are as follows:
name
description
price
The methods are as follows:
set_name()
get_name()
set_description()
get_description()
set_price()
get_price()
We will continue to learn as we work through these examples and we will also continue to refine this to make it even better. We need to build our foundations of understanding first. Now, we will look at an example of this in the following code:
<cfcomponent> <cffunction name="init"> <cfscript> variables.attributes = structNew(); variables.attributes.name = ""; variables.attributes.description = ""; variables.attributes.price = ""; </cfscript> <cfreturn this> </cffunction> </cfcomponent>
All this gives us to start with is what is called a constructor. This concept is common to most objects you will see in any language. You can see here that we store the actual name, description, and price attributes inside the object. You will also learn that things stored inside the "variables" of a CFC cannot be accessed outside of a CFC. When we first create an object, we call the constructor to construct the object.
It should be noted that the "variables" scope persists from one call to the next call of a CFC object. When we use variables that should exist only for the current function call in a CFC, all of the non-persistent variables should be declared with var
before the variable. This is not a var and a period, but a var and a space to declare that it is a local temporary variable.
Now that we have the object started, we are going to add what are called getters and setters. You can assume that they do exactly what you would guess. They get and set the attributes of an object. There are other ways to build objects that have various issues. We are teaching you a safe and professional way from the start. Now let us look at some more code. We will be adding these code lines right after the init
function/method we just put in our code. You will also note that we have added a ColdFusion comment to the page to help read through the code visually. You will also notice that if you are not writing multiple lines of code,<cfset>
is cleaner than wrapping stuff inside a<cfscript></cfscript>
tag pair.
Note
Make sure you save the CFC file as product_1.cfc
in the directory you are working in, for this chapter.
<cfcomponent> <cffunction name="init"> <cfscript> variables.attributes = structNew(); variables.attributes.name = ""; variables.attributes.description = ""; variables.attributes.price = ""; </cfscript> <cfreturn this> </cffunction> <!--- get/set attribute:name ---> <cffunction name="get_name"> <cfreturn variables.attributes.name> </cffunction> <cffunction name="set_name"> <cfargument name="name"> <cfset variables.attributes.name = arguments.name> </cffunction> <!--- get/set attribute:description ---> <cffunction name="get_description"> <cfreturn variables.attributes.description> </cffunction> <cffunction name="set_description"> <cfargument name="description"> <cfset variables.attributes.description = arguments.description> </cffunction> <!--- get/set attribute:price ---> <cffunction name="get_price"> <cfreturn variables.attributes.price> </cffunction> <cffunction name="set_price"> <cfargument name="price"> <cfset variables.attributes.price = arguments.price> </cffunction> </cfcomponent>
Now why would someone do this setter and getter thing? It is because this creates what is called "encapsulated variables". It takes a lot of experience before developers start to learn what type of simple things typically cause errors in code. This technique helps reduce that type of issue. We will take a look at the code in action before we get into telling you what it does. So save this file in a new directory for this chapter and create a file called product_1.cfc
.
Now we will create a standard ColdFusion page. Create a file called 2_1.cfm
and enter the following code. You can place your objects in a different file later, but for now make sure that all of your Chapter 2 files are in the same directory on your web server. You should also note that you refer to the filename without the .cfc
extension when creating the object instance. This is because when we set the component argument in the creation function, it assumes that on its own.
<!--- Example: 2_1.cfm ---> <!--- Processing ---> <cfscript> objProduct = createObject("component","product_1"); </cfscript> <!--- Content ---> <cfdump var="#objProduct#">
Here we have a page that creates what is called an "instance" of the object class. You can think of the actual CFC stored on the server as a pattern. Those patterns are called classes. ColdFusion uses the createObject
command to create an instance of the class. You can name the objects what you want and the name of the object does not have to match the name of the class you create the object from. We will prefix them with obj
right now just to help us remember that these are objects. Then we will dump it to the screen so we can get our first glimpse of what we are building.
Here we see that there are many defaults that are created for objects even if we don't declare them. Most of them are just empty and that is fine. You should also notice that the values passed into a method are called "arguments". Currently, our object has three methods. We are going to write an intentional bug into our software for just a moment so you can see what type of error occurs, for future reference. We will enhance our previous code, and remove the CFDump tag.
<!--- Example: 2_2.cfm ---> <!--- Processing ---> <cfscript> objProduct = createObject("component","product_1"); result = objProduct.get_name(); </cfscript> <!--- Content ---> <cfoutput> #result# </cfoutput>

Here is another nice thing about error messages when they work best. If you find that error messages don't point clearly to the problem, use CFDump or another debugging technique. The notice in the previous screenshot tells us that the problem is that variables.attributes.name doesn't seem to exist inside the CFC. Why is that?
The reason will become common and simple to you as you program. If you get errors that are obvious, consider yourself the same as every other developer. Don't let that make you think you are not a good or growing developer. It's just part of the process and you will enjoy how ColdFusion helps make the process easier.
The issue with this code is that we never called our constructor when we created our object instance. If the error is not as detailed, go to the ColdFusion Administrator, click on Debugging & Logging | Debug Output Settings, and select the Enable Robust Exception Information option. Save and reload the page.
Tip
A constructor is a method in an object class that lets us configure the object to its initial state for use.