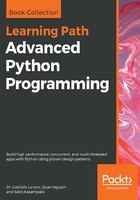
Classes
We can define an extension type using the cdef class statement and declaring its attributes in the class body. For example, we can create an extension type--Point--as shown in the following code, which stores two coordinates (x, y) of the double type:
cdef class Point
cdef double x
cdef double y
def __init__(self, double x, double y):
self.x = x
self.y = y
Accessing the declared attributes in the class methods allows Cython to bypass expensive Python attribute look-ups by direct access to the given fields in the underlying C struct. For this reason, attribute access in typed classes is an extremely fast operation.
To use the cdef class in your code, you need to explicitly declare the type of the variables you intend to use at compile time. You can use the extension type name (such as Point) in any context where you will use a standard type (such as double, float, and int). For example, if we want a Cython function that calculates the distance from the origin (in the example, the function is called norm) of a Point, we have to declare the input variable as Point, as shown in the following code:
cdef double norm(Point p):
return (p.x**2 + p.y**2)**0.5
Just like typed functions, typed classes have some limitations. If you try to access an extension type attribute from Python, you will get an AttributeError, as follows:
>>> a = Point(0.0, 0.0)
>>> a.x
AttributeError: 'Point' object has no attribute 'x'
In order to access attributes from Python code, you have to use the public (for read/write access) or readonly specifiers in the attribute declaration, as shown in the following code:
cdef class Point:
cdef public double x
Additionally, methods can be declared with the cpdef statement, just like regular functions.
Extension types do not support the addition of extra attributes at runtime. In order to do that, a solution is defining a Python class that is a subclass of the typed class and extends its attributes and methods in pure Python.