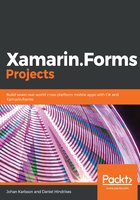
Defining a ViewModel base class
A ViewModel is the mediator between the View and the Model. We can benefit greatly by creating a common base class for all our ViewModels to inherit from. To do this, follow these steps:
- Create a folder called ViewModels in the DoToo .NET Standard project.
- Create a class called ViewModel in the ViewModels folder .
- Resolve references to System.ComponentModel and Xamarin.Forms and add the following code:
public abstract class ViewModel : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
public void RaisePropertyChanged(params string[] propertyNames)
{
foreach (var propertyName in propertyNames)
{
PropertyChanged?.Invoke(this, new
PropertyChangedEventArgs(propertyName));
}
}
public INavigation Navigation { get; set; }
}
The ViewModel class is a base class for all ViewModels. This is not meant to be instantiated on its own, so we mark it as abstract. It implements INotifyPropertyChanged, which is an interface defined in System.ComponentModel in the .NET base class libraries. This interface only defines one thing: the PropertyChanged event. Our ViewModel must raise this event whenever we want the GUI to be aware of any changes to a property. This can be done manually, by adding code to a setter in a property, or by using an intermediate language (IL) weaver such as PropertyChanged.Fody. We will talk about this in detail in the next section.
We are also taking a little shortcut here by adding an INavigation property in the ViewModel. This will help us with navigation later on. This is also something that can (and should) be abstracted, since we don't want the ViewModel to be dependent on Xamarin.Forms, in order to be able to reuse the ViewModels on any platform.