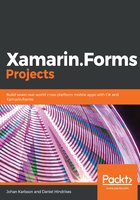
Creating the TodoItemViewModel
The TodoItemViewModel is the ViewModel that represents each item in the to-do list on the MainView. It will not have an entire view of its own (although it could have), but instead will be rendered by a template in the ListView. We will get back to this when we create the controls for the MainView.
The important thing here is that this ViewModel will represent a single item, regardless of where we choose to render it.
Let's create the TodoItemViewModel:
- Create a class called TodoItemViewModel inside the ViewModels folder.
- Add the following template code and resolve the references:
public class TodoItemViewModel : ViewModel
{
public TodoItemViewModel(TodoItem item) => Item = item;
public event EventHandler ItemStatusChanged;
public TodoItem Item { get; private set; }
public string StatusText => Item.Completed ? "Reactivate" :
"Completed";
}
As with any other ViewModel, we inherit the TodoItemViewModel from ViewModel. We conform to the pattern of injecting all dependencies in the constructor. In this case, we pass an instance of the TodoItem class in the constructor that the ViewModel will use to expose to the view.
The ItemStatusChanged event handler will be used later when we want to signal to the view that the state of the TodoItem has changed. The Item property allows us to access the item that we passed in.
The StatusText property is used for making the status of the to-do item human readable in the view.