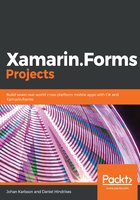
Creating the ItemView
Next up is the second view. We will use this for adding and editing to-do list items:
- Create a new Content Page (the same way as we created the MainView) and name it ItemView.
- Edit the XAML and make it look like the following code:
<?xml version="1.0" encoding="UTF-8"?>
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="DoToo.Views.ItemView"
Title="New todo item">
<ContentPage.ToolbarItems>
<ToolbarItem Text="Save" />
</ContentPage.ToolbarItems>
<StackLayout Padding="14">
<Label Text="Title" />
<Entry />
<Label Text="Due" />
<DatePicker />
<StackLayout Orientation="Horizontal">
<Switch />
<Label Text="Completed" />
</StackLayout>
</StackLayout>
</ContentPage>
As with the MainView, we need a title. We will give it a default title of "New todo item" for now, but we will change this to "Edit todo item" when we reuse this view for editing later on. The user must be able to save a new or edited item, so we have added a toolbar save button. The content of the page uses a StackLayout to structure the controls. A StackLayout adds an element vertically (the default option) or horizontally based on the space it calculates that the element takes up. This is a CPU-intensive process, so we should only use it on small portions of our layout. In the StackLayout, we add a Label that will be a line of text over the Entry control that comes underneath it. The Entry control is a text input control that will contain the name of the to-do list item. We then have a section for a DatePicker, where the user can select a due date for the to-do list item. The final control is a Switch control, which renders a toggle button to control when an item is completed, and a heading next to that. Since we want these to be displayed next to each other horizontally, we use a horizontal StackLayout to do this.
The last step for the views is to wire up the ItemViewModel to the ItemView:
- Open up the code-behind file of the ItemView by expanding the ItemView.xaml file in the Solution Explorer.
- Modify the constructor of the class to look like the following code. Add the code that is marked in bold.
- Add a using DoToo.ViewModels statement at the top of the following file the existing using statements:
public ItemView (ItemViewModel viewmodel)
{
InitializeComponent ();
viewmodel.Navigation = Navigation;
BindingContext = viewmodel;
}
This code is identical to the code that we added for MainView, except for the type of the ViewModel.