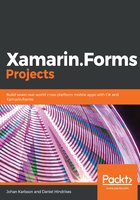
Navigating from the MainView to the ItemView to add a new item
We have an Add toolbar button in the MainView. When the user taps this button, we want to navigate to the ItemView. The MVVM way to do this is to define a command and then bind that command to the button. Let's add the code:
- Open ViewModels/MainViewModel.cs.
- Add using statements for System.Windows.Input, DoToo.Views, and Xamarin.Forms.
- Add the following property to the class:
public ICommand AddItem => new Command(async () =>
{
var itemView = Resolver.Resolve<ItemView>();
await Navigation.PushAsync(itemView);
});
All commands should be exposed as a generic ICommand. This abstracts the actual command implementation, which is a good general practice to follow. The command must be a property; in our case, we are creating a new Command object that we assign to this property. The property is read-only, which is usually fine for a Command. The action of the command (the code that we want to run when the command is executed) is passed to the constructor of the Command object.
The action of the command creates a new ItemView through the Resolver and Autofac builds the necessary dependencies. Once the new ItemView has been created, we simply tell the Navigation service to push it onto the stack for us.
After that, we just have to wire up the AddItem command from the ViewModel to the add button in the view:
- Open Views/MainView.xaml.
- Add the Command attribute to the ToolbarItem:
<ContentPage.ToolbarItems>
<ToolbarItem Text="Add" Command="{Binding AddItem}" />
</ContentPage.ToolbarItems>
Run the app and tap the Add button to navigate to the new item view. Notice that the back button appears automatically.