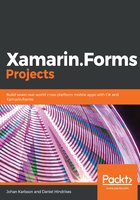
Binding the ListView in the MainView
A to-do list is not much use without a list of items. Let's extend the MainViewModel with a list of items:
- Open ViewModels/MainViewModel.cs.
- Add using statements for System.Collections.ObjectModel and System.Linq.
- Add a property for the to-do list items:
public ObservableCollection<TodoItemViewModel> Items { get; set; }
An ObservableCollection is like an ordinary collection, but it has a useful superpower. It can notify listeners about changes in the list, such as when items are added or deleted. The Listview will listen to changes in the list and update itself automatically based on these.
We now need some data:
- Open ViewModels/MainViewModel.cs.
- Replace (or complete) the LoadData method and create the CreateTodoItemViewModel and ItemStatusChanged methods.
- Resolve the reference to DoToo.Models by adding a using statement:
private async Task LoadData()
{
var items = await repository.GetItems();
var itemViewModels = items.Select(i =>
CreateTodoItemViewModel(i));
Items = new ObservableCollection<TodoItemViewModel>
(itemViewModels);
}
private TodoItemViewModel CreateTodoItemViewModel(TodoItem item)
{
var itemViewModel = new TodoItemViewModel(item);
itemViewModel.ItemStatusChanged += ItemStatusChanged;
return itemViewModel;
}
private void ItemStatusChanged(object sender, EventArgs e)
{
}
The LoadData method calls the repository to fetch all items. We then wrap each to-do list item in the TodoItemViewModel. This will contain more information that is specific to the view and that we don't want to add to the TodoItem class. It is a good practice to wrap plain objects in a ViewModel; this makes it simpler to add actions or extra properties to it. The ItemStatusChanged is a stub that will be called when we change the status of the to-do list item from active to completed and vice versa.
We also need to hook up some events from the repository to know when data changes:
- Open ViewModels/MainViewModel.cs.
- Add the following code in bold:
public MainViewModel(TodoItemRepository repository)
{
repository.OnItemAdded += (sender, item) =>
Items.Add(CreateTodoItemViewModel(item));
repository.OnItemUpdated += (sender, item) =>
Task.Run(async () => await LoadData());
this.repository = repository;
Task.Run(async () => await LoadData());
}
When an item is added to the repository, no matter who added it, the MainView will add it to the items list. Since the items collection is an observable collection, the list will update. If an item gets updated, we simply reload the list.
Let's data-bind our items to the ListView:
- Open up MainView.xaml and locate the ListView element.
- Modify it to reflect the following code:
<ListView Grid.Row="1"
RowHeight="70"
ItemsSource="{Binding Items}">
<ListView.ItemTemplate>
<DataTemplate>
<ViewCell>
<Grid Padding="15,10">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="10" />
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<BoxView Grid.RowSpan="2" />
<Label Grid.Column="1"
Text="{Binding Item.Title}"
FontSize="Large" />
<Label Grid.Column="1"
Grid.Row="1"
Text="{Binding Item.Due}"
FontSize="Micro" />
<Label Grid.Column="1"
Grid.Row="1"
HorizontalTextAlignment="End"
Text="Completed"
IsVisible="{Binding Item.Completed}"
FontSize="Micro" />
</Grid>
</ViewCell>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
The ItemsSource binding tells the ListView where to find the collection to iterate over and is local to the ViewModel. Any bindings inside the ViewCell node, however, are local to each item that we iterate in the list. In this case, we are binding to the TodoItemViewModel, which contains a property named Item. This, in turn, has properties such as Title, Due, and Completed. We can navigate down the hierarchy of objects without any problem when defining a binding.
The DataTemplate defined what each row will look like. We use a grid to partition the space just like we did earlier.