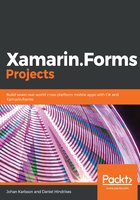
Marking an item as complete using a command
We need to add a functionality that allows us to toggle the items between complete and active. It is possible to navigate to the detailed view of the to-do list item, but this is too much work for a user. Instead, we'll add a ContextAction to the ListView. In iOS, for example, this will be accessed by swiping left on a row:
- Open ViewModel/TodoItemViewModel.cs.
- Add a using statement for System.Windows.Input and Xamarin.Forms.
- Add a command to toggle the status of the item and a piece of text that describes the status:
public ICommand ToggleCompleted => new Command((arg) =>
{
Item.Completed = !Item.Completed;
ItemStatusChanged?.Invoke(this, new EventArgs());
});
Here, we have added a command for toggling the state of an item. When executed, it inverses the current state and raises the ItemStatusChanged event so that subscribers are notified. To change the text of the context action button depending on the status, we added a StatusText property. This is not a recommended practice, because we are adding code that only exists because of a specific UI case into the ViewModel. Ideally, this would be handled by the view, perhaps by using a ValueConverter. To save us having to implement these steps, however, we have left it as a string property:
- Open Views/MainView.xaml.
- Locate the ListView.ItemTemplate node and add the following ViewCell.ContextActions node:
<ListView.ItemTemplate>
<DataTemplate>
<ViewCell>
<ViewCell.ContextActions>
<MenuItem Text="{Binding StatusText}"
Command="{Binding ToggleCompleted}" />
</ViewCell.ContextActions>
<Grid Padding="15,10">
...
</Grid>
</DataTemplate>
</ListView.ItemTemplate>