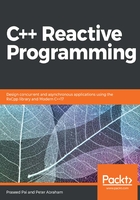
Substitutability
In the previous example, we saw how a user-defined type can be used to express all the operations done on a built-in type. Another goal of C++ is to write code in a generic manner where we can substitute a user-defined class that mimics the semantics of one of the built-in types such as float, double, int, and so on:
//------------- from SmartValue.cpp
template <class T>
T Accumulate( T a[] , int count ) {
T value = 0;
for( int i=0; i<count; ++i) { value += a[i]; }
return value;
}
int main(){
//----- Templated version of SmartFloat
SmartValue<double> y[] = { 10,20.0,30,40 };
double res = Accumulate(y,4);
cout << res << endl;
}
The C++ programming language supports different programming paradigms and the three principles outlined previously are just some of them. The language gives support for constructs that can help create robust types (domain-specific) for writing better code. These three principles gave us a powerful and fast programming language for sure. Modern C++did add a lot of new abstractions to make the life of a programmer easier. But the three design principles outlined previously have not been sacrificed in any way to achieve those objectives. This was partly possible because of the meta programming support the language had due to the inadvertent Turing completeness of the template mechanism. Read about template meta programming (TMP) and Turing Completeness with the help of your favorite search engine.