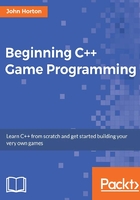
C++ strings
In the previous chapter we briefly mentioned strings and we learned that a string can hold alphanumeric data in anything from a single character to a whole book. We didn't look at declaring, initializing, or manipulating strings. So let's do that now.
Declaring strings
Declaring a string variable is simple. We state the type, followed by the name:
String levelName; String playerName;
Once we have declared a string we can assign a value to it.
Assigning a value to strings
To assign a value to a string, as with regular variables, we simply put the name, followed by the assignment operator, then the value:
levelName = "Dastardly Cave"; playerName = "John Carmack";
Note that the values need to be enclosed in quotation marks. As with regular variables we can also declare and assign values in a single line:
String score = "Score = 0"; String message = "GAME OVER!!";
This is how we can change our string variables.
Manipulating strings
We can use the #include <sstream>
directive to give us some extra power with our strings. The sstream
class enables us to add some strings together. When we do so it is called concatenation:
String part1 = "Hello "; String part2 = "World"; sstream ss; ss << part1 << part2; // ss now holds "Hello World"
In addition to using sstream
objects, a string variable can even be concatenated with a variable of a different type. This next code starts to reveal how strings might be quite useful to us:
String scoreText = "Score = "; int score = 0; // Later in the code score ++; sstream ss; ss << scoreText << score; // ss now holds "Score = 1"
Tip
The <<
operator is a bitwise operator. C++ however, allows you to write your own classes and override what a specific operator does, within the context of your class. The sstream
class has done this to make the <<
operator work the way it does. The complexity is hidden in the class. We can use its functionality without worrying about how it works. If you are feeling adventurous you can read about operator overloading at: http://www.tutorialspoint.com/cplusplus/cpp_overloading.htm. You don't need any more information in order to continue with the project.
Now we know the basics of C++ strings, and how we can use sstream
, we can see how to use some SFML classes to display them on the screen.