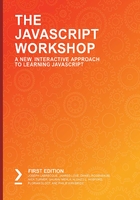
Syntax
Programs follow a set of rules that define keywords, symbols, and structure. This is called the syntax. We have already learned about many of the syntax rules in JavaScript for expressing data, variables, and expressions. You will be required to name objects, properties, methods, variables, and functions.
The following is a basic set of rules and conventions. Conventions are another term for best practices. Although not following a convention will not cause issues in your coding, they can make your code less easy to follow and not palatable to other programmers, for example, who may be interviewing you for a job and ask to see your code samples.
The naming rules and conventions for functions and variables are as follows:
- 26 upper and lowercase letters (A-Z, a-z).
- Any character but the first character can be one of 10 digits (0-9).
- No spaces, dashes, or commas. The underscore (_) character is acceptable.
- Capitalization follows camelCase. This means that all characters are lowercase, except for the first letters of words and except for the first word in compound worded names.
- No JavaScript reserved words; for example, you cannot use typeof, var, let, and const.
Semicolon at the End of Code Statements
Some programming languages require a semicolon ; at the end of every executable code statement. JavaScript does not have this requirement, except when you have more than one executable code statement on the same line of a JavaScript file.
Requiring a semicolon ; at the end of every executable code statement is more of a personal or development team choice. Since the semicolon character ; is used in other languages, often, programmers prefer to use them in JavaScript so that they get into the habit of using them and so that they spend less time dealing with syntax errors. If you choose to use the semicolon character ;, then do it consistently.
Lines of Code versus Statements
Each line in a JavaScript source file does not need to be a single line of executable code. You can break a single line of executable code into multiple source file lines, or put multiple lines of executable code on a single source file line. This flexibility allows you to format the code so that it is easier to follow and edit.
The following is a single line of executable code using a single source file line:
let todoList = ["Laundry", "Letters", "Groceries", "Mail", "Dinner"]
However, it may be more desirable to use multiple source file lines, like so:
let todoList = [
"Laundry",
"Letters",
"Groceries",
"Mail",
"Dinner"
]
You can have more than one line of code on the same line if you use ; after the previous code line:
var bid = 10; checkBid(bid)l
You will learn that, when JavaScript files are prepared for publishing, you can optionally use an optimizer program to compress all the lines in a source file into one line. In this way, the invisible end of a line character is removed to make the file smaller.
Comments
You can add a comment to your code since they are ignored when the program is executed. Comments can help us remember what the code does at a future date and inform other programmers who may need to use or work with your code.
Commenting is a useful tool to keep a line of code from executing in testing. For example, let's say you have one or more lines of code that are not working as expected and you want to try alternative code. You can comment on the code in question while you try the alternatives.
JavaScript has inline commenting, also known as single-line commenting. This uses the double forward slash, //. All the text following the double slash up to the end of the line is ignored when the program is executed.
Let's have a look at some examples of inline comments. The following comment explains the next line of code:
// Hide all result message elements
matchedMsgEle.style.display = 'none';
The comment at the end of the line is explaining the code:
let numberGuessed = parseInt(guessInputEle.value); // NaN or integer
JavaScript has block commenting, also known as multi-line commenting. This uses the combined forward slash asterisk characters to mark the beginning of the comment, and the reverse of a combined asterisk forward-slash to mark the end of the comment. All the text between /* and */ is ignored when the program is executed. Let's have a look at the various block comments.
The following is a multiple-line block comment that contains code. This code snippet would not be executed:
/* This is a block comment.
It can span multiple lines in the file.
Code in a comment is ignored such as the next line.
var profit = revenue - cost;
*/
A block comment can be a single line in the file:
/* This is a block comment. */
The following is an example of using JDoc block comments for a function:
/**
* Shuffles array elements
* @param {array} sourceArray - Array to be shuffled.
* @returns {array} - New array with shuffled items
*/
function getNewShuffledArray(sourceArray){
// Statements for function
}
There are also tools that use syntax to produce documentation of your code from comments (for example, JDoc). These tools read your source code and produce a documentation guide of your code. Comments increase the bandwidth for web pages, so they are often not seen in the source code when you inspect a web page. This is because, often, the original JavaScript file is not published, but rather a compressed version. The tools that compress the JavaScript file will remove comments by default. Comments are helpful for learning. You are encouraged to write comments in your code that explain what the code does.