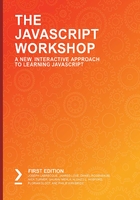
Conditional and Loop Flow
Statements in JavaScript are processed sequentially in the order they're loaded. That order can be changed with conditional and loop code statements. The different parts of a control statement are as follows:
- Code blocks {…}
- Conditional flow statements, such as if...else, switch, try catch finally
- Loop statements, such as for, do...while, while, for...in, and for...of
- Other control statements, such as labeled, break, and continue
We will describe each of these in detail in the next section.
Code Blocks
Code blocks are statements that are placed between an open and close curly bracket. The syntax is as follows:
//Code block
{
//Statement
//Statement
//Statement
}
Code blocks by themselves do not offer any statement flow advantage until you combine them with conditional or loop statements.
Conditional Flow Statements
Conditional statements use logic expressions to choose from among a set of statements to process.
if...else Statement
The if, else...if, and else statements give you four structures for selecting or skipping blocks of code.
if Statement
Code in an if statement is processed if the expression evaluates to true and is skipped if the expression evaluates to false. The syntax is as follows:
if(boolean expression){
//Statement
//Statement
//Statement
}
if(boolean expression)
//Single statement
This shows the flow of the if statement. If the Boolean expression is true, the code is processed. If false, the code is skipped:

Figure 3.4: The if flowchart
Exercise 3.05: Writing an if statement
In this exercise, you will use the if statement to test for an even number between 1 and 6 and test the results in your web browser console window. Let's get started:
- Open the if-statement.html document in your web browser.
- Open the web developer console window using your web browser.
- Open the if-statement.js document in your code editor, replace all of its content with the following code, and then save it:
var diceValue = Math.floor(Math.random() * 6) + 1;
console.log("Dice value:", diceValue);
if(diceValue % 2 != 0){
console.log("Is an odd number.");
}
- The Math.random() function randomly creates a whole number from 1 to 6 and displays it in the console. Here, the if statement states that if the remainder of the number, divided by two, is not zero, that is, diceValue % 2 != 0, then the if expression is true and the console.log() message is displayed in the console.
- Reload the if-statement.html web page in your web browser with the console window open. Repeat until you see a version of the two examples:
// Example of output if the number is odd.
Dice value: 3
Is an odd number.
// Example of output if the number is even.
Dice value: 4
- Edit the if-statement.js document using bolded lines and then save it:
var diceValue = Math.floor(Math.random() * 10) + 1;
console.log("Dice value:", diceValue);
console.log("Is an odd number.");
}
Because there is only one line of code in the if statement, the block brackets are not required.
- Reload the if-statement.html web page in your web browser with the console window open. You should expect the same results.
- Edit the if-statement.js document and add the highlighted line to console.log() and save it:
var diceValue = Math.floor(Math.random() * 6) + 1;
console.log("Dice value:", diceValue);
if(diceValue % 2 != 0)
console.log("Is an odd number.");
console.log('"You have to be odd to be number one", Dr. Seuss');
- Reload the if-statement.html web page in your web browser with the console window open:
// Example of output if the number is odd.
Dice value: 3
Is an odd number.
"You have to be odd to be number one", Dr. Seuss
// Example of output if the number is even.
Dice value: 2
"You have to be odd to be number one", Dr. Seuss
The Dr. Seuss quote is shown regardless of whether the number is even or odd.
- Edit the if-statement.js document lines in bold and save it. We added the block delimiters here:
console.log("Is an odd number.");
console.log('"You have to be odd to be number one", Dr. Seuss');
}
- Reload the if-statement.html web page in your web browser with the console window open:
// Example of output if the number is odd. The Dr. Seuss quote is included when the value is an odd number.
Dice value: 3
Is an odd number.
"You have to be odd to be number one", Dr. Seuss
// Example of output if the number is even. The Dr. Seuss quote is skipped when the value is an even number.
Dice value: 2
You can see different outcomes depending on the logical expression of the if statement.
if Statement and else Statement
You can combine an if statement with an else statement. If the expression evaluates to true, the code in the if statement is processed and the code in the else statement is skipped. If the expression is false, the reverse happens; that is, the code in the if statement is skipped and the code in the else statement is processed. The syntax is as follows:
if(boolean expression){
//Statement
//Statement
//Statement
}else{
//Statement
//Statement
//Statement
}
if(boolean expression)
//Single statement
else
//Single statement
The if...else working is visible from the following flowchart:

Figure 3.5: The if else flowchart
Exercise 3.06: Writing an if...else Statement
In this exercise, a random number is being used for a coin toss. A random value equal to .5 or greater is heads and less than .5 is tails. We will assume that multiple statement lines are required for each case. Let's get started:
- Open the if-else-statements.html document in your web browser.
- Open the web developer console window using your web browser.
- Open the if-else-statements.js document in your code editor, replace all of its content with the following code, and then save it:
var tossValue = Math.random();
console.log("Random toss value:", tossValue);
if(tossValue>= .5){
console.log("Heads");
}
The tossValue variable is a value from 0 to 1, not including 1. For now, just an else statement is used for a head toss.
- Reload the if-else-statements.html web page in your web browser with the console window open. Repeat until you see a version of the two examples:
// Example of output if the number is .5 or greater.
Random toss value: 0.8210720135035767
Heads
// Example of output if the number is less than .5.
Random toss value: 0.4565522878478414
//random()gives out a different value each time
Note
The numbers that you obtain are likely to be different to the ones presented here.
- Edit the if-else-statements.js document, add the following bolded code, and then save it:
if(tossValue>= .5){
console.log("Heads");
}else{
console.log("Tails");
}
If the if statement expression is true, the statements in its block are processed and the else block statements are skipped. If the if block expression is false, only the statements in the else block are processed.
- Reload the if-else-statements.html web page in your web browser with the console window open:
// Example of output if the number is .5 or greater.
Random toss value: 0.9519471939452648
Heads
// Example of output if the number is less than .5.
Random toss value: 0.07600044264786021
Tails
Again, you will see different outcomes depending on the logical expression of the if statement. Consider how an if statement may handle toggling a like icon on the screen.
if Statements with Multiple else...if Statements
You can have one or more else...if statements in addition to the if statement. The if statement and each else...if statement has its own expression. If the code in the first statement has an expression evaluated as true, it is processed and the code in all the other statements is skipped. If none of the expressions evaluate to true, all the code statements are skipped. The syntax is as follows:
if(boolean expression){
//Statement
//Statement
//Statement
}else if(boolean expression){
//Statement
//Statement
//Statement
}else if(boolean expression){
//Statement
//Statement
//Statement
}
if(boolean expression)
//Single statement
else if(boolean expression)
//Single statement
else if(boolean expression)
//Single statement
The following flowchart illustrates one or more else...if statements in addition to the if statement. Each of the Boolean expressions is evaluated in the order they're encountered. The code is processed if the first expression is true, and the code processing procedure skips to the code following the last else...if statement:

Figure 3.6: The if and multiple else...if flowchart
if Statement, Multiple else...if statements, and the else Statement
You can have one else statement follow the last else...if statement. If the code in the first statement has an expression evaluated to true, is it processed and the code in all other statements is skipped. If none of the expressions evaluate to true, then the code in the else statement is processed. The syntax is as follows:
if(boolean expression){
//Statement
//Statement
//Statement
}else if(boolean expression){
//Statement
//Statement
//Statement
}else if(boolean expression){
//Statement
//Statement
//Statement
}else{
//Statement
}
if(boolean expression)
//Single statement
else if(boolean expression)
//Single statement
else if(boolean expression)
//Single statement
else
//Single statement
The following flowchart the illustrates inclusion of the else statement, along with else if statements and the if statement. If all the Boolean expressions are false, then the code in the else block is processed:

Figure 3.7: The else statement, along with else...if statements and the if statement
Exercise 3.07: Writing an if Statement with Multiple if else Statements and the else Statement
In this exercise, we will build a simple game that generates four random game numbers from 1 to 21 inclusive. One is the player's score, one is the target score, one is a lucky score, and the last is an unlucky score. The player gets a wallet of 20 times the player's score. There are five possible outcomes, with each assigning different wins or losses to the player's wallet:
- The player's score matches the lucky score, and the lucky score and the unlucky score are different. The wallet is increased by the lucky value plus the player's score times 10.
- The player's score equals the unlucky score, and the lucky score and the unlucky score are different. The wallet is reduced to zero.
- The player's score equals the target score. The wallet is increased by the difference between 21 and the target score times 10.
- The player's score beats the target score. The wallet is increased by the difference between the player's score and the target score times 10.
- The target score beats the player's score. The wallet is decreased by the difference between the target score and the player's score times 10.
The steps for completion are as follows:
- Open the if-else-if-else-statements.html document in your web browser.
- Open the web developer console window using your web browser.
- Open the if-else-if-else-statements.js document in your code editor, replace all of its content with the following code, and then save it:
var target = Math.floor(Math.random() * 21) + 1;
var player = Math.floor(Math.random() * 21) + 1;
console.log("Target score:", target);
console.log("Player score:", player);
if (player >= target){
console.log("Player wins: beats target by " + (player - target));
}else{
console.log("Player loses: misses target by " + (target - player));
}
We will start by matching the target or exceeding it using the if statement block, if (player >= target). The else statement block stating "Player loses: misses target by" covers being below the target.
- Reload the if-else-if-else-statements.html web page in your web browser with the console window open. Repeat until you see a version for each of these three examples.
An example of the player's score exceeding the target is as follows:
Target score: 5
Player score: 13
Player wins: beats target by 8
The following is an example of the player's score matching the target. In this case, the message is not supporting the logic:
Target score: 14
Player score: 14
Player wins: beats target by 0
An example of the target exceeding the player's score is as follows:
Target score: 19
Player score: 1
Player loses: misses target by 18
Now, we can add a code some handle the player matching the target.
- Edit the if-else-if-else-statements.js document, add the following bolded code, and then remove the strikethrough code and save it:
console.log("Player score:", player);
if (player == target){
console.log("Player wins: ties target " + target);
}else if (player > target){
console.log("Player wins: beats target by " + (player - target);
}else{
A new if statement block is added to handle the condition when the player ties with the target. The original if statement block is replaced with an else...if statement block that only tests for conditions when the player's value exceeds the target.
- Reload the if-else-if-else-statements.html web page in your web browser with the console window open. Repeat this until you see a version for each of these three examples.
An example of a player's score exceeding the target is as follows:
Target score: 7
Player score: 14
Player wins: beats target by 7
The following is an example of a player matching the target. In this case, the message is not supporting the logic:
Target score: 3
Player score: 3
Player wins: ties target 3
An example of the target exceeding the player's score is as follows:
Target score: 10
Player score: 5
Player loses: misses target by 5
- Edit the if-else-if-else-statements.js document, update it using the following bolded code, and then save it.
A variable for the lucky and unlucky number are added and are output to the console so that we can observe them:
var target = Math.floor(Math.random() * 21) + 1;
var player = Math.floor(Math.random() * 21) + 1;
var lucky = Math.floor(Math.random() * 21) + 1;
var unlucky = Math.floor(Math.random() * 21) + 1;
console.log("Target score:", target);
console.log("Player score:", player);
console.log("Lucky score:", lucky);
console.log("Unlucky score:", unlucky);
- Next, we add an if statement block when the lucky value does not match the unlucky value and the player value matches the lucky value. The use of the logical && operator handles the two required tests, both of which need to be true.
This condition preempts the other winning and losing condition if statements, so it needs to precede them. Add the following bolded code and remove the strikethrough code:
if (lucky != unlucky && player == lucky){
console.log("Player wins: matches lucky score.");
}else if (player == target){
console.log("Player wins: ties target " + target);
}
- We also want a condition when the lucky value does not match the unlucky value, and the player value matches the unlucky value. Again, the use of the logical && operator handles the two required tests, both of which need to be true.
This condition preempts the other winning and losing condition if statements, so it needs to precede them. Insert the following bolded code:
if (lucky != unlucky && player == lucky){
console.log("Player wins: matches lucky score.");
}else if (lucky != unlucky && player == unlucky){
console.log("Player loses: matches unlucky score.");
}else if (player == target){
- Reload the if-else-if-else-statements.html web page in your web browser with the console window open. Repeat this until you see a version for each of these two examples.
The following is an example of a player matching the lucky number, but not the unlucky number:
Target score: 7
Player score: 14
Lucky score: 16
Unlucky score: 20
Player wins: matches lucky score
The following is an example of a player matching the unlucky number, but not the lucky number:
Target score: 4
Player score: 9
Lucky score: 3
Unlucky score: 9
Player loses: matches unlucky score.
- Edit the if-else-if-else-statements.js document, update it with the following bolded code, and then save it.
The initial wallet value is 10 times the player's score. It is displayed along with the other game data:
var unlucky = Math.floor(Math.random() * 21) + 1;
var wallet = player * 20;
console.log("Target score:", target);
console.log("Unlucky score:", unlucky);
console.log("Player initial wallet:", wallet);
If there is a match with the lucky number, the wallet is increased by the player's score and the lucky score times 10.
if (lucky != unlucky && player == lucky){
console.log("Player wins: matches lucky score.");
wallet += (lucky + player) * 10;
If there is a match with the unlucky number, the wallet is decreased to zero:
}else if (lucky != unlucky && player == unlucky){
console.log("Player loses: matches unlucky score.");
wallet = 0;
If the player's score matches the target, the wallet is increased by the difference between 21 and the target:
}else if (player == target){
console.log("Player wins: ties target " + target);
wallet += (21 - target) * 10;
If the player's score exceeds the target, the wallet is increased by the difference times 10:
}else if (player > target){
console.log("Player wins: beats target by " + (player - target));
wallet += (player - target) * 10;
The else statement block reduces the wallet by the difference between the target and the player. It ties 10, but not below zero.
After the if, if else, and else block statements, the player's final wallet is displayed:
}else{
console.log("Player loses: misses target by " + (target - player));
wallet = Math.max(0, wallet - (target - player) * 10);
}
console.log("Player final wallet:", wallet);
- Reload the if-else-if-else-statements.html web page in your web browser with the console window open. Repeat this until you see a version for each of these examples.
The following is an example of the target exceeding the player's score and the amount being deducted from the wallet exceeding the wallet balance. In this case, the wallet is reduced to zero:
Target score: 4
8 Player score: 1
Lucky score: 6
Unlucky score: 4
Player initial wallet: 20
Player loses: misses target by 3
Players final wallet: 0
The following is an example of the player's score exceeding the target score. The wallet increased by 10 times the difference exceeded:
Target score: 10
Player score: 18
Lucky score: 21
Unlucky score: 10
Player initial wallet: 360
Player wins: beats target by 8
Players final wallet: 440
The following is an example of the player's score matching the target score. The wallet increased by 10 times the difference of 21 and the target:
Target score: 19
Player score: 19
Lucky score: 4
Unlucky score: 7
Player initial wallet: 380
Player wins: ties target 19
Players final wallet: 400
The following is an example of the player matching the lucky number, but not the unlucky number. The wallet is increased by the player and the target times 10:
Target score: 19
Player score: 1
Lucky score: 1
Unlucky score: 7
Player initial wallet: 20
Player wins: matches lucky score.
Players final wallet: 40
The following is an example of the player matching the unlucky number, but not the lucky number. The wallet is reduced to 0:
Target score: 8
Player score: 13
Lucky score: 10
Unlucky score: 13
Player initial wallet: 260
Player loses: matches unlucky score.
Players final wallet: 0
This was a much longer exercise. It showed you how multiple if statements with different logic expressions can work together to produce one outcome. You will have noticed that the order of the logical expressions can make a difference because in this case, the lucky and unlucky values needed to be resolved before the target value expressions. Changing the order would produce a whole set of different outcomes.
The break Statement
The break statement is used within blocks for loop statements and the switch statements. When the break statement is encountered inside loop statement and switch statement blocks, program flow continues on the next line following the block. The syntax is as follows:
break
break label
The second syntax form is required when it's used within a labeled statement block. You will find out more about labeled statements later in this chapter. The upcoming exercises will make use of the break statement.
switch Statement
The switch statement defines a block of code divided up by case statements and an optional default statement. The case statements are followed by a possible value for the switch statement expression and then a colon, :. Optionally, the code will follow a case statement. The default statement is just followed by a colon, :.
How does it work? The switch statement's expression is evaluated and the code following the first case statement that has a value that matches the switch statement's expression value is processed until a break statement is reached. Then, any remaining code is skipped. If none of the case statement values match and there is a default statement, then the code following the default statement is processed. Otherwise, no code is processed. The syntax is as follows:
switch(expression){
case expression_value:
//Optional statement(s)
break; //optional
case expression_value:
//Optional statement(s)
break; //optional
default:
//Statement(s)
}
The following is a flowchart illustrating the switch statement:

Figure 3.8: Switch statement flowchart
Exercise 3.08: Writing a switch Statement and Testing It
We are going to use the switch statement by simulating a game where the player can move their playing pieces using their keyboard. They can move left with the A key, right with the S key, up with the W key, and down with the Z key. To simulate a random selection of the keys, in either uppercase or lowercase, from the keyNames string, a variable will be used. Let's get started:
- Open the switch-statement.html document in your web browser.
- Open the web developer console window using your web browser.
- Open the switch-statement.js document in your code editor, replace all of its content with the following code, and then save it:
var keyNames = "WASDwasd";
var keyName = keyNames.charAt(Math.floor(Math.random() * keyNames.length));
console.log("keyName:", keyName);
The Math.floor(Math.random() * keys.length) expression is selecting a number from 0 to 7 that is then used by charAt to select the character from the keyNames string variable.
- Run a few tests by reloading the switch-statement.html web page in your web browser with the console window open. Your results will show selections from the ADWSadws characters. Here are some examples of the console output:
keyName: a
keyName: S
- Edit the switch-statement.js document so that it includes the following bolded lines and then save it.
The switch statement expression is as follows:
console.log("keyName:", keyName);
switch (keyName.toLowerCase()){
The switch statement expression converts the character into lowercase so that each case statement can check for one value. Here, we are checking whether the case value is equal to the switch term:
case "a":
console.log("move left"); //This block will execute when break; // keyName is a
case "d":
console.log("move right");//This block will execute when break; // keyName is d
case "w":
console.log("move up");//This block will execute when break; // keyName is w
case "s":
console.log("move down");//This block will execute when break; // keyName is s
}
The switch statement uses one expression and then determines the lines of code to process based on matching the result with the case statements. It is important to note that without a break statement, all the code to the end of the switch statement is processed once one case statement matches the expression value. This can be an advantage when more than one case statement uses the same code. The default statement allows for code that is being processed when none of the case statements match the expression value. However, remember that a default statement is not required. In this example, if the user pressed a wrong key, nothing would happen, which is often the case with game consoles.
- Reload the switch-statement.html web page in your web browser with the console window open. The following are some sample results:
keyName: S
move down
keyName: d
move right
Let's use the IJKL keys to perform the same tasks. We'll use the I key for up, the J key for left, the K key for right, and the M key for down.
Edit the switch-statement.js document, include the following bolded lines and save it.
First, add the new key letters:
var keyNames = "WASDwasdIJKMijkm";
Next, add the case statements for each:
case "a":
case "j":
console.log("move left");
break;
case "d":
case "k":
console.log("move right");
break;
case "w":
case "i":
console.log("move up");
break;
case "s":
case "m":
console.log("move down");
break;
}
When case statements are not followed by a break, the next case statement's code is also processed.
- Reload the switch-statement.html web page in your web browser with the console window open. The following are some sample results:
keyName: J
move left
keyName: w
move up
The simulated code does not generate any keys that are not matched by the case statements. If there were, the entire switch statement is skipped. The switch statement can handle other cases by using the default statement.
- Edit the switch-statement.js document, include the following bolded lines, and then save it. First, let's add a few test characters:
var keyNames = "WASDwasdIJKMijkmRTXPrtxp";
Next, let's add the default statement:
case "m":
console.log("move down");
break;
default:
console.log("invalid key");
break;
}
- Reload the switch-statement.html web page in your web browser with the console window open. Repeat this until you see a result indicating an invalid key:
keyName: R
invalid key
In this exercise, if the user pressed a wrong key, nothing would happen, which is often the case with game consoles.
Loop Statements
Loop code blocks are also called iterative blocks. They are designed to continue processing the code in their blocks until the loop statement expression becomes false. Iteration is a term that's used to indicate one time through the loop.
Note
A loop that does not terminate is called an infinite loop. A web browser may display a dialog with the option to terminate long-running loops.
for Statement
The for statement repeats the code until the repeat expression becomes false. The syntax is as follows:
for(initialize statement; repeat expression; post expression){
//Statement
//Statement
}
The following flowchart depicts how the for statement works:

Figure 3.9: for statement flowchart
The first time the for statement is reached, the initialize statement is processed. It normally sets a variable that is used in the repeat expression. The post expression changes a value in the repeat expression. After the last line of code in the loop is processed, the post expression is processed and then the repeat expression is processed. If the repeat expression remains true, the first statement in the loop block is processed again. The post expression often makes use of the arithmetic operators called increment and decrement and the assignment addition and subtraction operators. Some examples of more arithmetic operators are as follows:

Figure 3.10: More arithmetic operators
Exercise 3.09: Writing a for Loop and Testing It
This exercise demonstrates using the for statement to create an increasing counter and decreasing counter. Let's get started:
- Open the for-statement.html document in your web browser.
- Open the web developer console window using your web browser.
- Open the for-statement.js document in your code editor, replace all of its content with the following code, and then save it:
for(var i = 1; i<= 5; i++){
console.log(i);
}
This example is an incrementing counter loop. The initialize statement declares the i variable and assigns it a value of 1. This is the value it has on the first iteration of the loop. At the end of the loop, the repeat expression is evaluated and, if true, the line following the loop is processed. The post expression uses the increment operator to increase the i 1 variable at the end of each loop.
- Reload the for-statement.html web page in your web browser with the console window open. The following are the results:
1
2
3
4
5
- Edit the for-statement.js document using the following bolded lines and then save it:
for(var i = 5; i>= 1; i--){
console.log(i);
}
This example illustrates a decreasing counter loop. In this example, the post expression uses the decrement operator. The repeat expression is changed to be true until the i variable values are 1 or less. The initialize statement declares the i variable and sets it to 5.
- Reload the for-statement.html web page in your web browser with the console window open. The following are the results:
5
4
3
2
1
- Edit the for-statement.js document using the following bolded lines and then save it:
for(var i = 2; i<= 10; i+=2){
console.log(i);
}
This example features the addition assignment operator is used to create a counter loop that increments by 2, starting with 2 and ending with 10.
- Reload the for-statement.html web page in your web browser with the console window open. The following are the results:
2
4
6
8
10
The for loop is the workhorse for repeating code for a counted number of iterations. You will find greater use for it by iterating through arrays.
do...while Statement
The do...while statement is a loop that executes code until the repeat expression value becomes false. The repeat expression is evaluated after all the statements have been processed, resulting in the guarantee that they are processed once. The syntax is as follows:
do{
//Statement
//Statement
} while(repeat expression
do
//Single statement
while(repeat expression)
A semicolon needs to be at the end of the while line if you are using it elsewhere.
Here is the flowchart for the do…while statement:

Figure 3.11: do...while statement flowchart
Exercise 3.10: Writing a do...while Loop and Testing It
In this exercise, you will use the do…while loop to simulate iterating the roll of two dice until they have the same value. Let's get started:
- Open the do-while-statements.html document in your web browser.
- Open the web developer console window using your web browser.
- Open the do-while-statements.js document in your code editor, replace all of its content with the following code, and then save it:
do{
var die1 = Math.floor(Math.random() * 6) + 1;
var die2 = Math.floor(Math.random() * 6) + 1;
console.log("Die 1:", die1, "Die 2:", die2);
}while(die1 != die2);
The second and third lines each compute a random number from 1 to 6 and store it in a variable. Those variables are displayed on the third line. These lines are always executed once. The while condition is true if the values of the die1 and die2 variables are not equal. If the values are equal, the expression is false and the loop repeats. If the values are not equal, any statements that follow the do…while loop are processed.
- Run a few tests by reloading the do-while-statements.html web page in your web browser with the console window open. Your results will differ due to the random values.
The following is an example result of more than one iteration:
Die 1: 1 Die 2: 3
Die 1: 2 Die 2: 3
Die 1: 3 Die 2: 4
Die 1: 4 Die 2: 5
Die 1: 3 Die 2: 3
The following example shows the results of a single iteration. do…while loop statements are always processed one at a time:
Die 1: 5 Die 2: 5
- Edit the do-while-statements.js document so that it includes the following bolded lines and then save it:
let iterations = 0;
do{
iterations++;
var die1 = Math.floor(Math.random() * 6) + 1;
var die2 = Math.floor(Math.random() * 6) + 1;
console.log("Die 1:", die1, "Die 2:", die2);
}while(die1 != die2);
console.log("The matched value is: ", die1);
console.log("Number of iterations: ", iterations);
The first line, let iterations, is declaring a variable named iterations and assigning it to 0. Then, in the do…while loop, the iterations variable, iterations++, is incremented by 1. After the loop, the matched value and the iterations are displayed.
- Run a few tests by reloading the do-while-statements.html web page in your web browser with the console window open. Your results will differ due to the random values.
The following example is the result of more than one iteration:
Die 1: 1 Die 2: 3
Die 1: 2 Die 2: 3
Die 1: 5 Die 2: 4
Die 1: 3 Die 2: 1
Die 1: 4 Die 2: 4
The matched value is: 4
Number of iterations: 5
The following example is the result of a single iteration:
Die 1: 4 Die 2: 4
The matched value is: 4
Number of iterations: 1
while Statement
The while statement is a loop that executes code if the repeat expression is true/false. The repeat expression is evaluated before any code is executed, so there is the possibility that no code is processed if it is false the first time round. The syntax is as follows:
while(repeat expression){
//Statement
//Statement
}
while (repeat expression)
//Single statement
The while statement flow is illustrated as follows:

Figure 3.12: Code statements in the while block
Exercise 3.11: Writing a while Loop and Testing It
In this exercise, we will use the while loop to simulate how many dice rolls it takes to roll an even number. Let's get started:
- Open the while-statement.html document in your web browser.
- Open the web developer console window using your web browser.
- Open the while-statement.js document in your code editor, replace all of its content with the following code, and then save it:
let iterations = 0;
while (iterations <10){
console.log("iterations:", iterations);
iterations ++;
}
This is just the initial shell for a while loop that repeats 10 times. The while loop's repeat expression is true if the iterations variable is below the value of 10. The first time the expression is evaluated, the iterations variable is 0. Inside the while loop, the iterations variable is incremented by 1 on the first line and will increase from 0 to 9 on each iteration of the loop.
- Reload the while-statement.html web page in your web browser with the console window open.
The results show the iterations variable increasing from 0 to 9 for 10 iterations:
iterations: 0
iterations: 1
iterations: 2
iterations: 3
iterations: 4
iterations: 5
iterations: 6
iterations: 7
iterations: 8
iterations: 9
- Edit the while-statement.js document using the following bolded lines and then save it. This adds the line to show a dice roll for each iteration:
die = Math.floor(Math.random() * 6) + 1;
console.log("die:", die);
iterations ++;
- Reload the while-statement.html web page in your web browser with the console window open.
- You will see a list of 10 dice values. Your values will differ:
die: 2
die: 5
die: 2
die: 4
die: 2
die: 3
die: 4
die: 2
die: 6
die: 1
- Edit the while-statement.js document using the following bolded lines and then save it.
This adds an if block to test for an even number of the dice roll. If true, the break statement terminates the while loop and the line following it is processed. The two lines following the while loop display how many iterations occurred and the value of the dice roll for that iteration:
let die;
while (iterations <10){
die = Math.floor(Math.random() * 6) + 1;
if (die % 2 == 0){
break;
}
iterations ++;
}
console.log("Number of iterations: ", iterations + 1);
console.log("Die value: ", die);
- Run a few tests by reloading the while-statement.html web page in your web browser with the console window open:
Number of iterations: 1
Die value: 2
The while-loop use a Boolean expression to determine whether any iterations of the code it contains occurred. In this case, if the iterations variable was greater than 10, no iterations would have occurred.
for...in Statement
The for...in statement allows us to iterate over an object data type. The variable in the for expression holds one of the names of the name-value pairs of the object, which are the names of the properties and methods of the object. The syntax is as follows:
for (variable in object){
//Statement
//Statement
}
for (variable in object)
//Single statement
You can declare the variable with const, var, or let.
Exercise 3.12: Writing a for...in Loop and Testing It
This exercise applies the for...in loop to the ready-made location object and to a programmer-created object. You can access object names and values by using them. Let's get started:
- Open the for-in-statement.html document in your web browser.
- Open the web developer console window using your web browser.
- Open the for-in-statement.js document in your code editor, replace all of its content with the following code, and then save it:
for (let name in location) {
console.log(name);
};
This iterates the web browser-created location object.
- Reload the for-in-statement.html web page in your web browser with the console window open.
The following output shows the names of all the location object's properties and methods:
replace
href
ancestorOrigins
origin
protocol
host
hostname
port
pathname
search
hash
assign
reload
toString
- Edit the for-in-statement.js document, add the following bolded text, and then save it:
for (let name in location) {
console.log(name, ":", location[name]);
};
This will add the value of the property or method.
- Reload the for-in-statement.html web page in your web browser with the console window open. The values may differ, assuming the web page was opened from a local file folder and not using http or https:
replace : ƒ () { [native code] }
href : file://PATH_TO/for-in-statement.html
ancestorOrigins :DOMStringList {length: 0}
origin : file://
protocol :
host :
hostname :
port :
pathname : /PATH_TO/for-in-statement.html
search :
hash :
assign : ƒ assign() { [native code] }
reload : ƒ reload() { [native code] }
toString : ƒ toString() { [native code] }
- Edit the for-in-statement.js document, replace it with the following code, and then save it:
var stopWatch = {
elapsedTime: 0,
resultsHistory: [],
isTiming: true,
isPaused: true,
start: function(){console.log("start");},
pause: function(){console.log("pause");},
resume: function(){console.log("resume");},
stop: function(){console.log("stop");}
};
- Below that, add the following code so that we can iterate through the object:
for (const name in stopWatch) {
console.log(name, ":", stopWatch[name]);
};
- Reload the for-in-statement.html web page in your web browser with the console window open.
The following is an example of the output in the console window:
elapsedTime : 0
resultsHistory : []
isTiming : true
isPaused : true
start : ƒ (){console.log("start");}
pause : ƒ (){console.log("pause");}
resume : ƒ (){console.log("resume");}
stop : ƒ (){console.log("stop");}
Looping through the methods and properties of objects can be helpful when the code depends on a specific property or name that needs to be present for it to work.
for...of Statement
The for...of statement focuses on iterable objects. Not all objects are iterable. Although we will not cover how to create our own iterable objects, there are some ready-made iterable objects that you may find the for∙∙∙of block useful for. The syntax is as follows:
for (variable of object){
//Statement
//Statement
}
for (variable of object)
//Single statement
You can declare the variable with const, var, or let.
Exercise 3.13: Writing a for...of Loop and Testing It
This exercise uses the for...of statement, which is designed for iterable objects. You will learn how some objects may not be iterable objects and generate errors. For iterable objects, arrays and strings are used. Let's get started:
- Open the for-of-statement.html document in your web browser.
- Open the web developer console window using your web browser.
- Open the for-of-statement.js document in your code editor, replace all of its content with the following code, and then save it:
var stopWatch = {
elapsedTime: 0,
resultsHistory: [],
isTiming: true,
isPaused: true,
start: function(){console.log("start");},
pause: function(){console.log("pause");},
resume: function(){console.log("resume");},
stop: function(){console.log("stop");}
};
- Below that, add the following code so that we can iterate through the object:
for (let name of stopWatch) {
console.log(name, ":", stopWatch[name]);
};
- Reload the for-of-statement.html web page in your web browser with the console window open.
An error will occur. We need to code the object so that it's iterable for it to work; however, we are not learning how to do that at this point:
Uncaught TypeError: stopWatch is not iterable
- Edit the for-of-statement.js document in your code editor, replace all of its content with the following code, and then save it.
A string turns out to be iterable:
let anyString = 'abcxyz123';
for (const value of anyString) {
console.log(value);
}
- Reload the for-of-statement.html web page in your web browser with the console window open:
a
b
c
x
y
z
1
2
3
- Edit the for-of-statement.js document in your code editor, make the changes shown in bold in the following code, and then save it.
A string turns out to be iterable:
let anyString = 'abcxyz123';
/*
for (let value of anyString) {
console.log(value);
}
*/
for (var i = 0; i<anyString.length; i++) {
console.log(anyString.charAt(i));
}
- Reload the for-of-statement.html web page in your web browser with the console window open. You will get the same results. The advantage of the for of loop is that it is more streamlined:
a
b
c
x
y
z
1
2
3
- Edit the for-of-statement.js document in your code editor, replace all of its content with the following code, and then save it.
An array is iterable:
let bowlingScores = [150, 160, 144, 190, 210, 185];
for (const value of bowlingScores) {
console.log(value);
}
- Reload the for-of-statement.html web page in your web browser with the console window open:
150
160
144
190
210
185
- Edit the for-of-statement.js document in your code editor, make the changes shown in bold in the following code, and then save it.
An array is iterable:
let bowlingScores = [150, 160, 144, 190, 210, 185];
/*
for (const value of bowlingScores) {
console.log(value);
}
*/
for (var i = 0; i<bowlingScores.length; i++) {
console.log(bowlingScores[i]);
}
- Reload the for-of-statement.html web page in your web browser with the console window open. You will get the same results:
150
160
144
190
210
185
Looping through the methods and properties of objects can be helpful when the code depends on a specific property or name that needs to be present for it to work.
continue Statement
The continue statement stops execution inside a loop or a labeled loop for the current iteration and starts the execution of the next loop iteration. The loop statements then determine whether another iteration should occur. The syntax is as follows:
continue
continue label
The second syntax is for use within a labeled statement block. We will learn more about labeled statements later in this chapter.
Labeled Statement
The Labeled statement is used to create loop flows and conditional flows. It names either block statements or loop statements. The syntax is as follows:
label : {
//Statement
//Statement
}
label : loop statement
When a loop statement is named, the statements are processed until a break statement or continue statement is encountered inside the block that references the label.
When a break statement is encountered, the program flow continues on the line after the labeled statement block referenced by the break statement. If a continue statement is encountered, the program flow continues on the first line of the block referenced by the continue statement. A continue statement requires the labeled statement to be a loop. Both break statements and continue statements must appear within the labeled statement block that they reference. They cannot appear outside the labeled statement block that they reference. They can appear in nested labeled blocks and reference outer labeled blocks. Labeled statements are less commonly used because they are prone to creating confusing or difficult to follow program flow.
Note
It is good practice to avoid or find ways to eliminate all labeled statements from code. Conditional statements and dividing code into functions or object methods are alternatives to labeled statements.
Let's have a look at an example of using a labeled loop statement. The loop labels the for statement, which runs 10 iterations. Each iteration generates a random number from 1 to 12. If the number is even, the continue statement starts the beginning of the for statement:
console.log("Odd number count started!");
let oddsCount = 0;
odd_number:
for (let i = 1; i<= 10; i++){
console.log("Iteration:", i);
var number = Math.floor(Math.random() * 12) + 1;
if (number % 2 == 0){
continue odd_number;
}
oddsCount ++;
console.log("Number:", number);
}
console.log(oddsCount + " odd numbers found!");
The output of the preceding code snippet is as follows:
Odd number count started!
Iteration: 1
Iteration: 2
Iteration: 3
Iteration: 4
Number: 7
Iteration: 5
Iteration: 6
Number: 5
Iteration: 7
Iteration: 8
Iteration: 9
Number: 3
3 odd numbers found!
The label can be eliminated with a better use of the if statement to achieve the same result:
console.log("Odd number count started!");
let oddsCount = 0;
for (let i = 1; i<= 10; i++){
console.log("Iteration:", i);
var number = Math.floor(Math.random() * 12) + 1;
if (number % 2 != 0){
oddsCount ++;
console.log("Number:", number);
}
}
console.log(oddsCount + " odd numbers found!");
The output is the same, but with different values:
Odd number count started!
Iteration: 1
Iteration: 2
Number: 9
Iteration: 3
Number: 5
Iteration: 4
Iteration: 5
Number: 5
Iteration: 6
Iteration: 7
Number: 9
Iteration: 8
Iteration: 9
Number: 1
Iteration: 10
Number: 7
6 odd numbers found!